Animated Heat Map Over Time
This is a random project “How to animate a heat map with date slider bar”
Lots of issues cropped up. Mostly from getting out of the swing of how to use pandas and different data structures/amending them when certain conditions are met.
This will be more useful once labels are added to the coordinates. I've worked in labels but it slows down Jupyter a lot and needs more work.
>>>Heat Map<<<from this CSV file
import folium
import folium.plugins as plugins
import numpy as np
from datetime import datetime, timedelta
import pandas as pd
# Read the data from the CSV file
df = pd.read_csv('data.csv')
# Convert the date columns to the appropriate data type
df['start_date'] = pd.to_datetime(df['start_date'])
df['end_date'] = pd.to_datetime(df['end_date'])
# Initialize a dictionary to store the data
data_dict = {}
# Iterate over each row in the DataFrame
for index, row in df.iterrows():
start_date = row['start_date']
end_date = row['end_date']
latitude = row['latitude']
longitude = row['longitude']
# Generate a list of dates between the start and end date
dates = pd.date_range(start=start_date, end=end_date, freq='D')
# Create a row for each date and append it to the data dictionary
for date in dates:
date_str = date.date().isoformat()
coordinates = [latitude, longitude]
if date_str in data_dict:
data_dict[date_str].append(coordinates)
else:
data_dict[date_str] = [coordinates]
# Convert the data dictionary to a list of lists
data = [[date, coordinates] for date, coordinates in data_dict.items()]
# Create a new DataFrame from the data list
new_df = pd.DataFrame(data, columns=['date', 'coordinates'])
date_list = new_df['date'].values.tolist()
coordinate_list = new_df['coordinates'].values.tolist()
# Save the DataFrame to a CSV file
new_df.to_csv('output.csv', index=False)
map_data = coordinate_list
time_index = date_list
m = folium.Map(location=[center_lat, center_lon], zoom_start=10)
hm = plugins.HeatMapWithTime(map_data, index=time_index, auto_play=True, max_opacity=.5, radius=30)
hm.add_to(m)
m.save('heatmap.html')
m
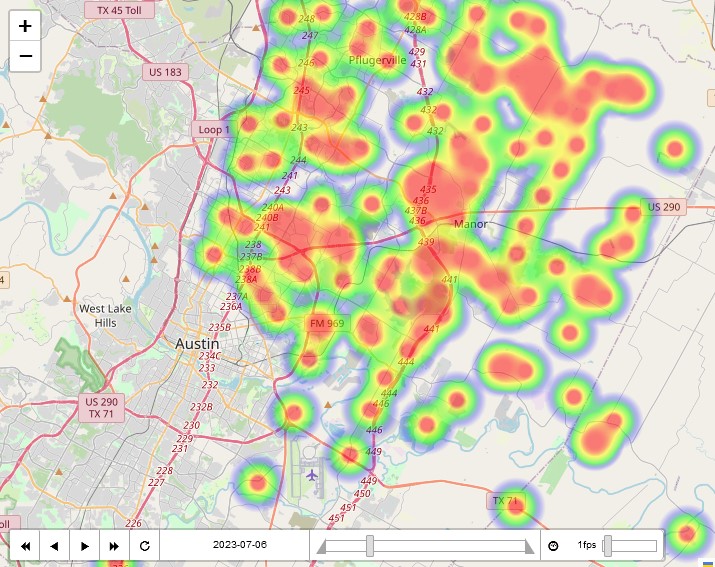